Helper Script: Event Logger/Listener
Post the following code snippet into your browser console
and then start triggering events by clicking around in your store.
Show Script
_65/**_65 * Custom Event Logger_65 * _65 * This script monitors and logs all custom events fired on any element._65 * It uses a MutationObserver to watch for new elements and attaches_65 * event listeners to both existing and newly added elements._65 */_65_65(function() {_65 // Store the original dispatchEvent method_65 const originalDispatchEvent = EventTarget.prototype.dispatchEvent;_65 _65 // Override the dispatchEvent method to intercept all event dispatches_65 EventTarget.prototype.dispatchEvent = function(event) {_65 // Only log custom events (those not starting with standard event prefixes)_65 const standardEventPrefixes = [_65 'mouse', 'pointer', 'key', 'touch', 'focus', 'blur', 'input', _65 'change', 'submit', 'scroll', 'resize', 'load', 'unload', 'DOMContent',_65 'animation', 'transition', 'drag', 'drop'_65 ];_65 _65 const isStandardEvent = standardEventPrefixes.some(prefix => _65 event.type.toLowerCase().startsWith(prefix.toLowerCase())_65 );_65 _65 if (!isStandardEvent) {_65 let targetInfo = '';_65 _65 // Try to get some identifying information about the target_65 if (this instanceof Element) {_65 targetInfo = this.tagName.toLowerCase();_65 _65 if (this.id) {_65 targetInfo += `#${this.id}`;_65 } else if (this.className && typeof this.className === 'string') {_65 targetInfo += `.${this.className.split(' ')[0]}`;_65 }_65 } else {_65 targetInfo = this.constructor.name || 'Unknown';_65 }_65 _65 // Log the event details_65 console.group(`%cCustom Event: ${event.type}`, 'color: #2196F3; font-weight: bold;');_65 console.log(`Target: ${targetInfo}`);_65 console.log('Event detail:', event.detail);_65 console.log('Full event object:', event);_65 console.log('Target:', this);_65 console.groupEnd();_65 }_65 _65 // Call the original method to maintain normal behavior_65 return originalDispatchEvent.call(this, event);_65 };_65 _65 console.log('%cCustom Event Logger activated', 'color: #4CAF50; font-weight: bold; font-size: 14px;');_65 console.log('Monitoring for custom events on all elements...');_65 _65 // Optional: Test the logger by dispatching a custom event_65 setTimeout(() => {_65 const testEvent = new CustomEvent('test-logger', { _65 detail: { message: 'This is a test custom event' } _65 });_65 document.dispatchEvent(testEvent);_65 }, 500);_65})();
Example Output
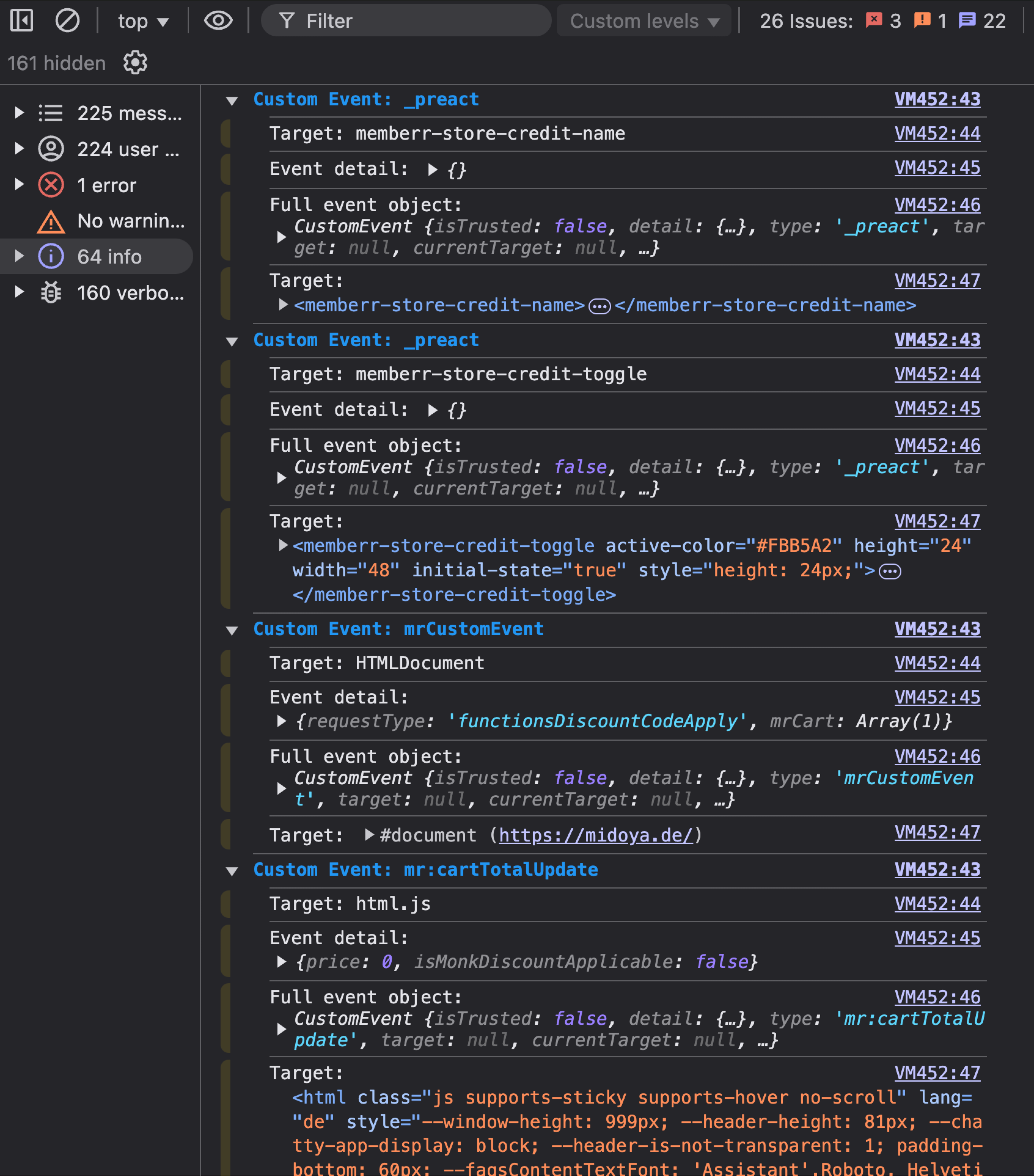